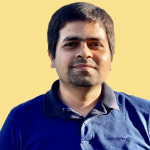
WWW.MARKTECHPOST.COM
A Coding Implementation for Building Python-based Data and Business intelligence BI Web Applications with Taipy: Dynamic Interactive Time Series Analysis, Real-Time Simulation, Seasonal Decomposition, and Advanced Visualization
In this comprehensive tutorial, we explore building an advanced, interactive dashboard with Taipy. Taipy is an innovative framework designed to create dynamic data-driven applications effortlessly. This tutorial will teach you how to harness its power to simulate complex time series data and perform real-time seasonal decomposition. By leveraging Taipy’s reactive state management, we construct a dashboard that allows seamless parameter adjustments, such as modifying trend coefficients, seasonal amplitudes, and noise levels, and updates visual outputs instantaneously. Integrated within a Google Colab environment, this tutorial provides a step-by-step approach to exploring the simulation and subsequent analysis using statsmodels, demonstrating how Taipy can streamline the development of rich, interactive visual analytics.
!pip install taipy statsmodels
We install the Taipy framework, which is essential for building interactive Python data and Business intelligence BI web applications. We also install stats models for sophisticated statistical analyses and time series decomposition. This setup ensures that all necessary libraries are available to run the advanced dashboard in Google Colab.
from taipy.gui import Gui
import numpy as np
import matplotlib.pyplot as plt
from statsmodels.tsa.seasonal import seasonal_decompose
We import the essential libraries to build an interactive dashboard with Taipy. It brings in Taipy’s Gui class for creating the web interface, NumPy for efficient numerical computations, matplotlib.pyplot for plotting graphs, and seasonal_decompose from statsmodels to perform seasonal decomposition on time series data.
state = {
"trend_coeff": 0.05,
"seasonal_amplitude": 5.0,
"noise_level": 1.0,
"time_horizon": 100,
"sim_data": None,
"times": None,
"plot_timeseries": None,
"plot_decomposition": None,
"summary": ""
}
We initialize a dictionary named state that serves as the reactive state container for the dashboard. Each key in the dictionary holds either a simulation parameter (like the trend coefficient, seasonal amplitude, noise level, and time horizon) or a placeholder for data and visual outputs (such as simulated data, time indices, the time series plot, the decomposition plot, and a summary string). This structured state allows the application to react to parameter changes and update the visualizations and analysis in real time.
def update_simulation(state):
t = np.arange(state["time_horizon"])
trend = state["trend_coeff"] * t
season = state["seasonal_amplitude"] * np.sin(2 * np.pi * t / 7)
noise = np.random.normal(0, state["noise_level"], size=state["time_horizon"])
sim_data = trend + season + noise
state["times"] = t
state["sim_data"] = sim_data
mean_val = np.mean(sim_data)
std_val = np.std(sim_data)
state["summary"] = f"Mean: {mean_val:.2f} | Std Dev: {std_val:.2f}"
fig, ax = plt.subplots(figsize=(8, 4))
ax.plot(t, sim_data, label="Simulated Data", color="blue")
ax.set_title("Simulated Time Series")
ax.set_xlabel("Time (days)")
ax.set_ylabel("Value")
ax.legend()
state["plot_timeseries"] = fig
try:
decomp = seasonal_decompose(sim_data, period=7)
fig_decomp, axs = plt.subplots(4, 1, figsize=(8, 8))
axs[0].plot(t, decomp.observed, label="Observed", color="blue")
axs[0].set_title("Observed Component")
axs[1].plot(t, decomp.trend, label="Trend", color="orange")
axs[1].set_title("Trend Component")
axs[2].plot(t, decomp.seasonal, label="Seasonal", color="green")
axs[2].set_title("Seasonal Component")
axs[3].plot(t, decomp.resid, label="Residual", color="red")
axs[3].set_title("Residual Component")
for ax in axs:
ax.legend()
fig_decomp.tight_layout()
state["plot_decomposition"] = fig_decomp
except Exception as e:
fig_err = plt.figure(figsize=(8, 4))
plt.text(0.5, 0.5, f"Decomposition Error: {str(e)}",
horizontalalignment='center', verticalalignment='center')
state["plot_decomposition"] = fig_err
This function, update_simulation, generates a synthetic time series by combining a linear trend, a sinusoidal seasonal pattern, and random noise. It then calculates summary statistics. It updates the reactive state with the simulation data. It produces two matplotlib figures, one for the simulated time series and one for its seasonal decomposition, while handling potential errors in the decomposition process.
update_simulation(state)
page = """
# Advanced Time Series Simulation and Analysis Dashboard
This dashboard simulates time series data using customizable parameters and performs a seasonal decomposition to extract trend, seasonal, and residual components.
## Simulation Parameters
Adjust the sliders below to modify the simulation:
- **Trend Coefficient:** Controls the strength of the linear trend.
- **Seasonal Amplitude:** Adjusts the intensity of the weekly seasonal component.
- **Noise Level:** Sets the randomness in the simulation.
- **Time Horizon (days):** Defines the number of data points (days) in the simulation.
<|{trend_coeff}|slider|min=-1|max=1|step=0.01|label=Trend Coefficient|on_change=update_simulation|>
<|{seasonal_amplitude}|slider|min=0|max=10|step=0.1|label=Seasonal Amplitude|on_change=update_simulation|>
<|{noise_level}|slider|min=0|max=5|step=0.1|label=Noise Level|on_change=update_simulation|>
<|{time_horizon}|slider|min=50|max=500|step=10|label=Time Horizon (days)|on_change=update_simulation|>
## Simulated Time Series
This plot displays the simulated time series based on your parameter settings:
<|pyplot|figure=plot_timeseries|>
## Seasonal Decomposition
The decomposition below splits the time series into its observed, trend, seasonal, and residual components:
<|pyplot|figure=plot_decomposition|>
## Summary Statistics
{summary}
---
*This advanced dashboard is powered by Taipy's reactive engine, ensuring real-time updates and an in-depth analysis experience as you adjust the simulation parameters.*
"""
Gui(page).run(state=state, notebook=True)
Finally, update_simulation(state) generates the initial simulation data and plots and defines a Taipy dashboard layout with interactive sliders, plots, and summary statistics. Finally, it launches the dashboard in notebook mode with Gui(page).run(state=state, notebook=True), ensuring real-time interactivity.
In conclusion, we have illustrated Taipy in creating sophisticated, reactive dashboards that bring complex data analyses to life. By constructing a detailed time series simulation paired with a comprehensive seasonal decomposition, we have shown how Taipy integrates with popular libraries like Matplotlib and statsmodels to deliver an engaging, real-time analytical experience. The ability to tweak simulation parameters on the fly and instantly observe their impact enhances understanding of underlying data patterns and exemplifies Taipy’s potential to drive deeper insights.
Here is the Colab Notebook. Also, don’t forget to follow us on Twitter and join our Telegram Channel and LinkedIn Group. Don’t Forget to join our 90k+ ML SubReddit.
Asif RazzaqWebsite | + postsBioAsif Razzaq is the CEO of Marktechpost Media Inc.. As a visionary entrepreneur and engineer, Asif is committed to harnessing the potential of Artificial Intelligence for social good. His most recent endeavor is the launch of an Artificial Intelligence Media Platform, Marktechpost, which stands out for its in-depth coverage of machine learning and deep learning news that is both technically sound and easily understandable by a wide audience. The platform boasts of over 2 million monthly views, illustrating its popularity among audiences.Asif Razzaqhttps://www.marktechpost.com/author/6flvq/OpenAI Releases Codex CLI: An Open-Source Local Coding Agent that Turns Natural Language into Working CodeAsif Razzaqhttps://www.marktechpost.com/author/6flvq/SQL-R1: A Reinforcement Learning-based NL2SQL Model that Outperforms Larger Systems in Complex Queries with Transparent and Accurate SQL GenerationAsif Razzaqhttps://www.marktechpost.com/author/6flvq/THUDM Releases GLM 4: A 32B Parameter Model Competing Head-to-Head with GPT-4o and DeepSeek-V3Asif Razzaqhttps://www.marktechpost.com/author/6flvq/Small Models, Big Impact: ServiceNow AI Releases Apriel-5B to Outperform Larger LLMs with Fewer Resources
0 Reacties
0 aandelen
34 Views