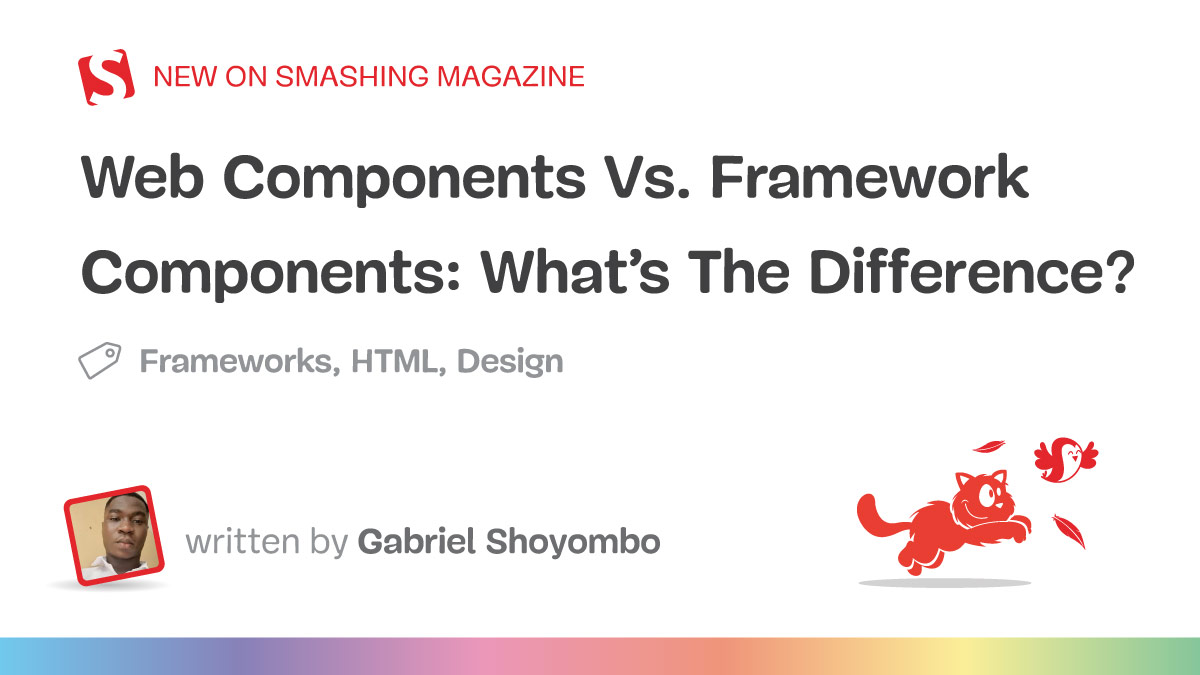
Web Components Vs. Framework Components:Whats The Difference?
smashingmagazine.com
It might surprise you that a distinction exists regarding the word component, especially in front-end development, where component is often used and associated with front-end frameworks and libraries. A component is a code that encapsulates a specific functionality and presentation. Components in front-end applications have a similar function: building reusable user interfaces. However, their implementations are different.Web or framework-agnostic components are standard web technologies for building reusable, self-sustained HTML elements. They consist of Custom Elements, Shadow DOM, and HTML template elements. On the other hand, framework components are reusable UIs explicitly tailored to the framework in which they are created. Unlike Web Components, which can be used in any framework, framework components are useless outside their frameworks.Some critics question the agnostic nature of Web Components and even go so far as to state that they are not real components because they do not conform to the agreed-upon nature of components. This article comprehensively compares web and framework components, examines the arguments regarding Web Components agnosticism, and considers the performance aspects of Web and framework components.What Makes A Component?Several criteria could be satisfied for a piece of code to be called a component, but only a few are essential:Reusability,Props and data handling,Encapsulation.Reusability is the primary purpose of a component, as it emphasizes the DRY (dont repeat yourself) principle. A component should be designed to be reused in different parts of an application or across multiple applications. Also, a component should be able to accept data (in the form of props) from its parent components and optionally pass data back through callbacks or events. Components are regarded as self-contained units; therefore, they should encapsulate their logic, styles, and state. If theres one thing we are certain of, framework components capture these criteria well, but what about their counterparts, Web Components?Understanding Web ComponentsWeb Components are a set of web APIs that allow developers to create custom, reusable HTML tags that serve a specific function. Based on existing web standards, they permit developers to extend HTML with new elements, custom behaviour, and encapsulated styling.Web Components are built based on three web specifications:Custom Elements,Shadow DOM,HTML templates.Each specification can exist independently, but when combined, they produce a web component. Custom ElementThe Custom Elements API makes provision for defining and using new types of DOM elements that can be reused.// Define a Custom Elementclass MyCustomElement extends HTMLElement { constructor() { super(); } connectedCallback() { this.innerHTML = ` <p>Hello from MyCustomElement!</p> `; }}// Register the Custom ElementcustomElements.define('my-custom-element', MyCustomElement);Shadow DOMThe Shadow DOM has been around since before the concept of web components. Browsers have used a nonstandard version for years for default browser controls that are not regular DOM nodes. It is a part of the DOM that is at least less reachable than typical light DOM elements as far as JavaScript and CSS go. These things are more encapsulated as standalone elements.// Create a Custom Element with Shadow DOMclass MyShadowElement extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); } connectedCallback() { this.shadowRoot.innerHTML = ` <style> p { color: green; } </style> <p>Content in Shadow DOM</p> `; }}// Register the Custom ElementcustomElements.define('my-shadow-element', MyShadowElement);HTML TemplatesHTML Templates API enables developers to write markup templates that are not loaded at the start of the app but can be called at runtime with JavaScript. HTML templates define the structure of Custom Elements in Web Components. // my-component.jsexport class MyComponent extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); } connectedCallback() { this.shadowRoot.innerHTML = ` <style> p { color: red; } </style> <p>Hello from ES Module!</p> `; }}// Register the Custom ElementcustomElements.define('my-component', MyComponent);<!-- Import the ES Module --><script type="module"> import { MyComponent } from './my-component.js';</script>Web Components are often described as framework-agnostic because they rely on native browser APIs rather than being tied to any specific JavaScript framework or library. This means that Web Components can be used in any web application, regardless of whether it is built with React, Angular, Vue, or even vanilla JavaScript. Due to their supposed framework-agnostic nature, they can be created and integrated into any modern front-end framework and still function with little to no modifications. But are they actually framework-agnostic?The Reality Of Framework-Agnosticism In Web ComponentsFramework-agnosticism is a term describing self-sufficient software an element in this case that can be integrated into any framework with minimal or no modifications and still operate efficiently, as expected.Web Components can be integrated into any framework, but not without changes that can range from minimal to complex, especially the styles and HTML arrangement. Another change Web Components might experience during integration includes additional configuration or polyfills for full browser support. This drawback is why some developers do not consider Web Components to be framework-agnostic. Notwithstanding, besides these configurations and edits, Web Components can easily fit into any front-end framework, including but not limited to React, Angular, and Vue.Framework Components: Strengths And LimitationsFramework components are framework-specific reusable bits of code. They are regarded as the building blocks of the framework on which they are built and possess several benefits over Web Components, including the following:An established ecosystem and community support,Developer-friendly integrations and tools,Comprehensive documentation and resources,Core functionality,Tested code,Fast development,Cross-browser support, andPerformance optimizations.Examples of commonly employed front-end framework elements include React components, Vue components, and Angular directives. React supports a virtual DOM and one-way data binding, which allows for efficient updates and a component-based model. Vue is a lightweight framework with a flexible and easy-to-learn component system. Angular, unlike React, offers a two-way data binding component model with a TypeScript focus. Other front-end framework components include Svelte components, SolidJS components, and more.Framework layer components are designed to operate under a specific JavaScript framework such as React, Vue, or Angular and, therefore, reside almost on top of the framework architecture, APIs, and conventions. For instance, React components use JSX and state management by React, while Angular components leverage Angular template syntax and dependency injection. As far as benefits, it has excellent developer experience performance, but as far as drawbacks are concerned, they are not flexible or reusable outside the framework.In addition, a state known as vendor lock-in is created when developers become so reliant on some framework or library that they are unable to switch to another. This is possible with framework components because they are developed to be operational only in the framework environment.Comparative AnalysisFramework and Web Components have their respective strengths and weaknesses and are appropriate to different scenarios. However, a comparative analysis based on several criteria can help deduce the distinction between both.Encapsulation And Styling: Scoped Vs. IsolatedEncapsulation is a trademark of components, but Web Components and framework components handle it differently. Web Components provide isolated encapsulation with the Shadow DOM, which creates a separate DOM tree that shields a components styles and structure from external manipulation. That ensures a Web Component will look and behave the same wherever it is used. However, this isolation can make it difficult for developers who need to customize styles, as external CSS cannot cross the Shadow DOM without explicit workarounds (e.g., CSS custom properties). Scoped styling is used by most frameworks, which limit CSS to a component using class names, CSS-in-JS, or module systems. While this dissuades styles from leaking outwards, it does not entirely prevent external styles from leaking in, with the possibility of conflicts. Libraries like Vue and Svelte support scoped CSS by default, while React often falls back to libraries like styled-components.Reusability And InteroperabilityWeb Components are better for reusable components that are useful for multiple frameworks or vanilla JavaScript applications. In addition, they are useful when the encapsulation and isolation of styles and behavior must be strict or when you want to leverage native browser APIs without too much reliance on other libraries. Framework components are, however, helpful when you need to leverage some of the features and optimisations provided by the framework (e.g., React reconciliation algorithm, Angular change detection) or take advantage of the mature ecosystem and tools available. You can also use framework components if your team is already familiar with the framework and conventions since it will make your development process easier.Performance ConsiderationsAnother critical factor in determining web vs. framework components is performance. While both can be extremely performant, there are instances where one will be quicker than the other.For Web Components, implementation in the native browser can lead to optimised rendering and reduced overhead, but older browsers may require polyfills, which add to the initial load. While React and Angular provide specific optimisations (e.g., virtual DOM, change detection) that will make performance improvements on high-flow, dynamic applications, they add overhead due to the framework runtime and additional libraries.Developer ExperienceDeveloper experience is another fundamental consideration regarding Web Components versus framework components. Ease of use and learning curve can play a large role in determining development time and manageability. Availability of tooling and community support can influence developer experience, too. Web Components use native browser APIs and, therefore, are comfortable to developers who know HTML, CSS, and JavaScript but have a steeper learning curve due to additional concepts like the Shadow DOM, custom elements, and templates that have a learning curve attached to them. Also, Web Components have a smaller community and less community documentation compared to famous frameworks like React, Angular, and Vue.Side-by-Side Comparison Web Components Benefits Framework Components Benefits Native browser support can lead to efficient rendering and reduced overhead. Frameworks like React and Angular provide specific optimizations (e.g., virtual DOM, change detection) that can improve performance for large, dynamic applications. Smaller bundle sizes and native browser support can lead to faster load times. Frameworks often provide tools for optimizing bundle sizes and lazy loading components. Leverage native browser APIs, making them accessible to developers familiar with HTML, CSS, and JavaScript. Extensive documentation, which makes it easier for developers to get started. Native browser support means fewer dependencies and the potential for better performance. Rich ecosystem with extensive tooling, libraries, and community support. Web Components Drawbacks Framework Components Drawbacks Older browsers may require polyfills, which can add to the initial load time. Framework-specific components can add overhead due to the frameworks runtime and additional libraries. Steeper learning curve due to additional concepts like Shadow DOM and Custom Elements. Requires familiarity with the frameworks conventions and APIs. Smaller ecosystem and fewer community resources compared to popular frameworks. Tied to the framework, making it harder to switch to a different framework. To summarize, the choice between Web Components and framework components depends on the specific need of your project or team, which can include cross-framework reusability, performance, and developer experience. ConclusionWeb Components are the new standard for agnostic, interoperable, and reusable components. Although they need further upgrades and modifications in terms of their base technologies to meet framework components standards, they are entitled to the title components. Through a detailed comparative analysis, weve explored the strengths and weaknesses of Web Components and framework components, gaining insight into their differences. Along the way, we also uncovered useful workarounds for integrating web components into front-end frameworks for those interested in that approach.ReferencesWhat are Web Components? (WebComponents.org)Web Components Specifications (WebComponents.org)Web Components (MDN)Using Shadow DOM (MDN)Web Components Arent Components, Keith J. Grant
0 Kommentare
·0 Anteile
·16 Ansichten